NSTextField控件应用详解
NSTextField用来接收用户文本输入,其可以接收键盘事件。创建NSTextFiled的示例代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| - (void)viewDidLoad { [super viewDidLoad]; _textField = [[NSTextField alloc]initWithFrame:NSMakeRect(50, 30, 200, 50)]; _textField.placeholderString = @"请填写你的梦想"; NSMutableAttributedString * attriString = [[NSMutableAttributedString alloc]initWithString:@"请填写你的梦想"]; [attriString addAttribute:NSForegroundColorAttributeName value:[NSColor redColor] range:NSMakeRange(5, 2)]; _textField.placeholderAttributedString = attriString; _textField.backgroundColor = [NSColor greenColor]; _textField.drawsBackground = YES; _textField.textColor = [NSColor blueColor]; _textField.bordered = YES; _textField.bezeled = YES; _textField.editable = YES; _textField.selectable = YES; _textField.bezelStyle = NSTextFieldSquareBezel; _textField.preferredMaxLayoutWidth = 100; _textField.maximumNumberOfLines = 5; [[_textField cell] setLineBreakMode:NSLineBreakByCharWrapping]; [[_textField cell]setUsesSingleLineMode:NO]; [[_textField cell] setTruncatesLastVisibleLine: YES ]; [self.view addSubview:_textField]; }
|
需要注意,在AppKit坐标体系中,原点在左下角,这和数学中的坐标系一致。运行工程,效果如下图所示:
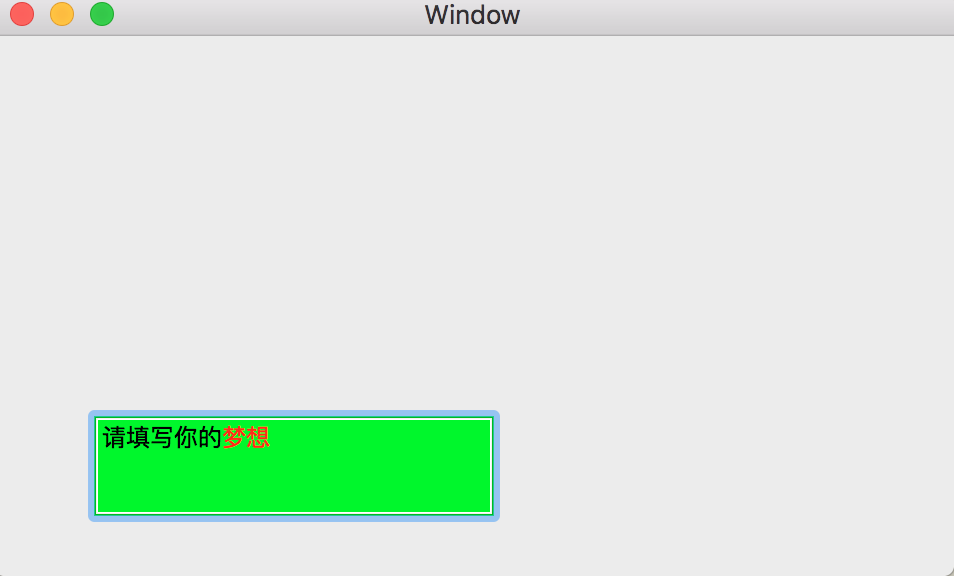
NSTextField类中常用的属性和方法列举如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| @property (nullable, copy) NSString *placeholderString NS_AVAILABLE_MAC(10_10);
@property (nullable, copy) NSAttributedString *placeholderAttributedString NS_AVAILABLE_MAC(10_10);
@property (nullable, copy) NSColor *backgroundColor;
@property BOOL drawsBackground;
@property (nullable, copy) NSColor *textColor;
@property (getter=isBordered) BOOL bordered;
@property (getter=isBezeled) BOOL bezeled;
@property (getter=isEditable) BOOL editable;
@property (getter=isSelectable) BOOL selectable;
@property (nullable, assign) id<NSTextFieldDelegate> delegate;
@property (readonly) BOOL acceptsFirstResponder;
@property NSTextFieldBezelStyle bezelStyle;
@property CGFloat preferredMaxLayoutWidth;
@property NSInteger maximumNumberOfLines;
@property BOOL allowsEditingTextAttributes;
@property BOOL importsGraphics;
- (void)selectText:(nullable id)sender;
- (BOOL)textShouldBeginEditing:(NSText *)textObject;
- (BOOL)textShouldEndEditing:(NSText *)textObject;
- (void)textDidBeginEditing:(NSNotification *)notification;
- (void)textDidEndEditing:(NSNotification *)notification;
- (void)textDidChange:(NSNotification *)notification;
@property (getter=isAutomaticTextCompletionEnabled) BOOL automaticTextCompletionEnabled NS_AVAILABLE_MAC(10_12_2);
@property BOOL allowsCharacterPickerTouchBarItem NS_AVAILABLE_MAC(10_12_2);
+ (instancetype)labelWithString:(NSString *)stringValue NS_SWIFT_NAME(init(labelWithString:)) NS_AVAILABLE_MAC(10_12); + (instancetype)wrappingLabelWithString:(NSString *)stringValue NS_SWIFT_NAME(init(wrappingLabelWithString:)) NS_AVAILABLE_MAC(10_12); + (instancetype)labelWithAttributedString:(NSAttributedString *)attributedStringValue NS_SWIFT_NAME(init(labelWithAttributedString:)) NS_AVAILABLE_MAC(10_12); + (instancetype)textFieldWithString:(nullable NSString *)stringValue NS_AVAILABLE_MAC(10_12);
|
NSTextField类继承自NSControl类,NSControl类中定义了许多属性可以获取到文本框中的文本,例如stringValue属性,本文中不再赘述。
关于NSTextFieldDelegate协议,其实际上是继承自NSControlTextEditingDelegate协议,这个协议中定义了NSTextField控件在活动过程中的回调方法,例如开始编辑,结束编辑等。