iOS开发之Accounts框架详解
Accounts框架是iOS原生提供的一套账户管理框架,其支持Facebook,新浪微博,腾讯微博,Twitter和领英账户管理的功能。需要注意,在iOS 11及以上系统中,将此功能已经删除,因此Accounts.framework实际上已经没有太大的意义,其只在iOS 11之前的系统上可用。
一、Accounts框架概览
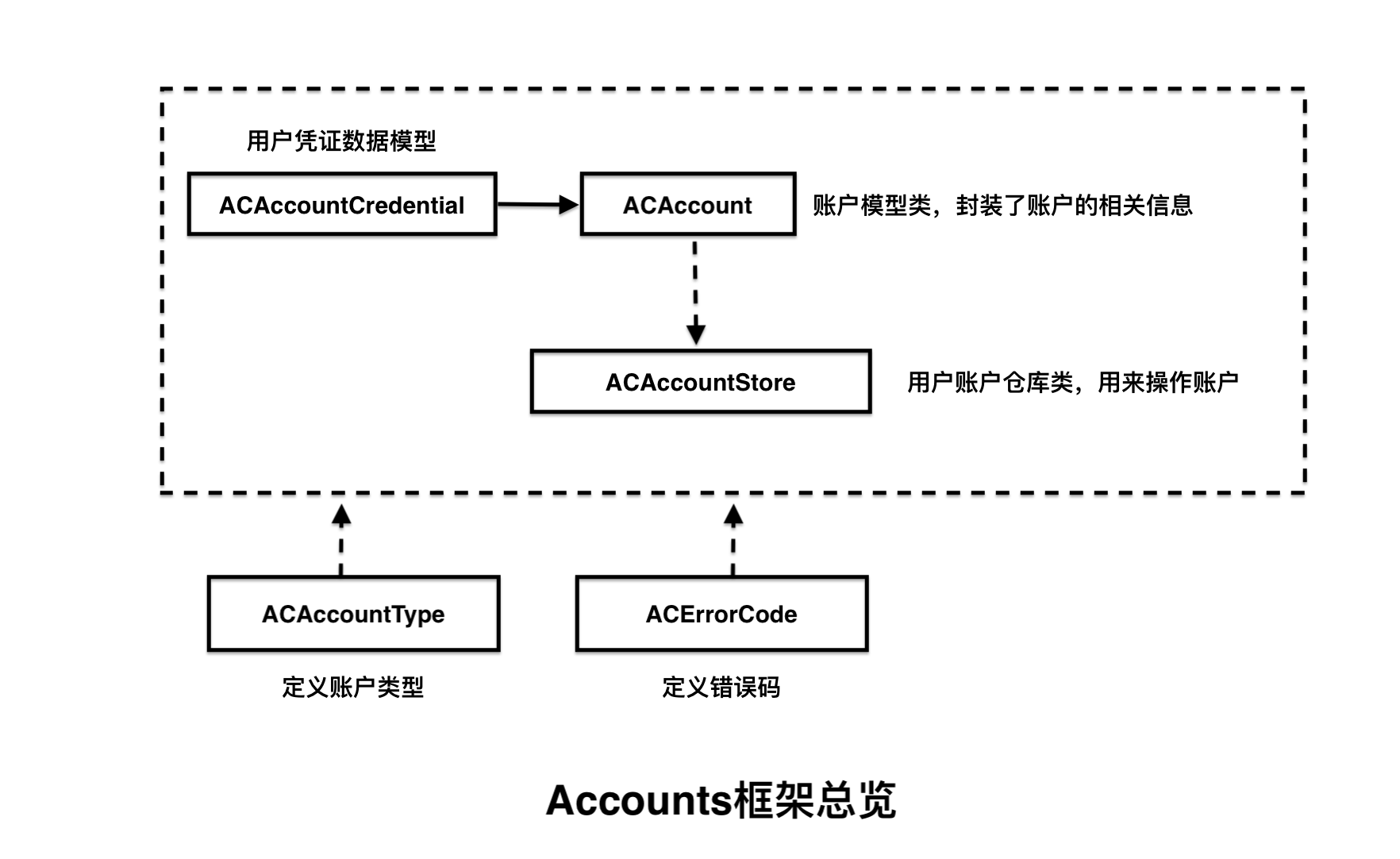
从上图可以看出,Accounts框架中最重要的3个类是ACAccountCredential类、ACAccount类和ACAccountStore类。后面我们着重介绍这3个类。
首先先来看ACAccountType类,这个类用来定义账户类型,如下:
1 2 3 4 5 6 7 8
| @interface ACAccountType : NSObject
@property (readonly, nonatomic) NSString *accountTypeDescription;
@property (readonly, nonatomic) NSString *identifier;
@property (readonly, nonatomic) BOOL accessGranted; @end
|
需要注意,这个类中的属性都是只读的,这也就是说,开发者不能够直接使用这个类进行对象的构建,需要借助ACAccountStore类来创建ACAccountType实例,后面会介绍。
ACErrorCode定义了错误码的意义,如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| typedef enum ACErrorCode { ACErrorUnknown = 1, ACErrorAccountMissingRequiredProperty, ACErrorAccountAuthenticationFailed, ACErrorAccountTypeInvalid, ACErrorAccountAlreadyExists, ACErrorAccountNotFound, ACErrorPermissionDenied, ACErrorAccessInfoInvalid, ACErrorClientPermissionDenied, ACErrorAccessDeniedByProtectionPolicy, ACErrorCredentialNotFound, ACErrorFetchCredentialFailed, ACErrorStoreCredentialFailed, ACErrorRemoveCredentialFailed, ACErrorUpdatingNonexistentAccount, ACErrorInvalidClientBundleID, ACErrorDeniedByPlugin, ACErrorCoreDataSaveFailed, ACErrorFailedSerializingAccountInfo, ACErrorInvalidCommand, ACErrorMissingTransportMessageID, ACErrorCredentialItemNotFound, ACErrorCredentialItemNotExpired, } ACErrorCode;
|
二、进行账户操作
在iOS 11以下的系统中,在设置中可以找到账户管理选项,如下图:
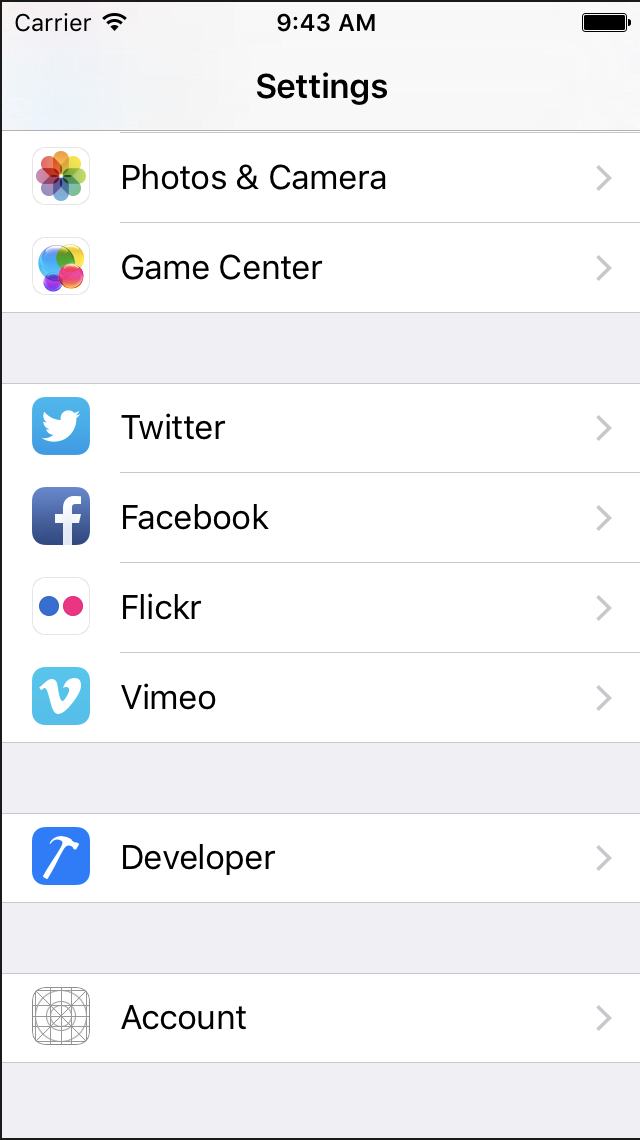
点击相应的社交平台,通过账号密码可以进行登录。这里一旦设置登录,那么在第三方应用程序中便可以通过Accounts框架来获取授权信息。
首先,要使用Accounts框架,需要导入相应头文件,如下:
1
| #import <Accounts/Accounts.h>
|
但应用程序首次使用用户社交平台的账户时,需要获取用户的授权,示例代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| ACAccountStore *accountStore = [[ACAccountStore alloc] init];
ACAccountType *accountType = [accountStore accountTypeWithAccountTypeIdentifier:ACAccountTypeIdentifierSinaWeibo];
[accountStore requestAccessToAccountsWithType: accountType options:nil completion:^(BOOL granted, NSError *error) { if (error) { NSLog(@"error = %@", [error localizedDescription]); } dispatch_async(dispatch_get_main_queue(), ^{ if(granted){ NSLog(@"授权通过了"); }); }];
|
获取用户授权界面如下图所示:
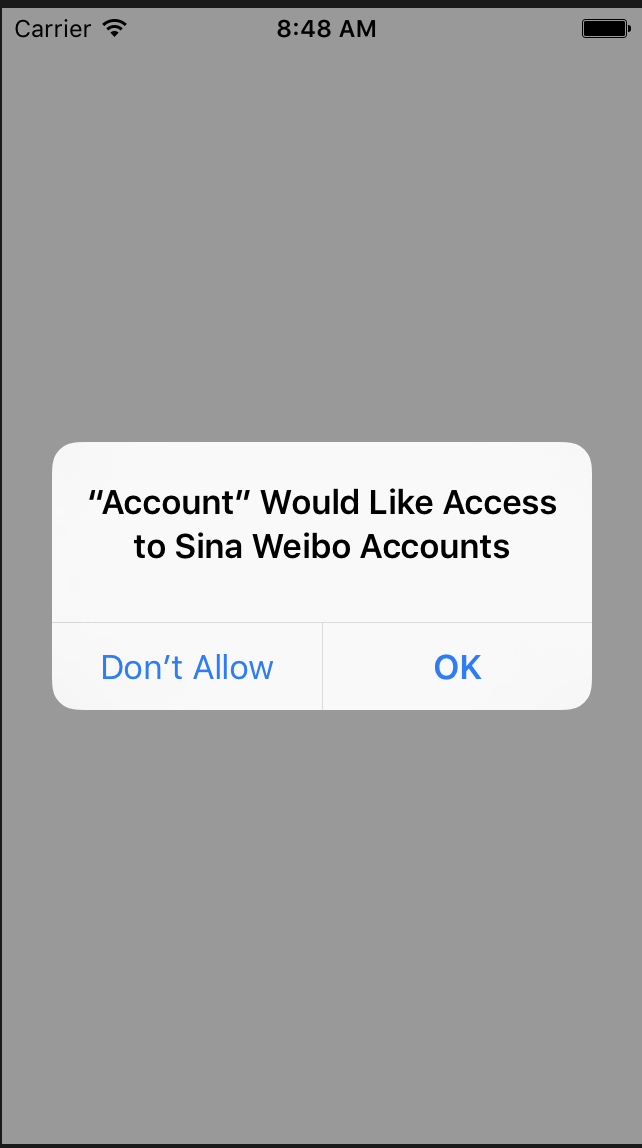
一旦用户授权通过,可以使用如下方法获取到用户的社交平台账户信息:
1 2 3
| ACAccountStore *accountStore = [[ACAccountStore alloc] init]; ACAccountType *accountType = [accountStore accountTypeWithAccountTypeIdentifier:ACAccountTypeIdentifierSinaWeibo]; NSArray *twitterAccounts = [accountStore accountsWithAccountType:accountType];
|
Accounts框架支持的社交平台类型ID如下:
1 2 3 4 5
| NSString * const ACAccountTypeIdentifierTwitter; NSString * const ACAccountTypeIdentifierFacebook; NSString * const ACAccountTypeIdentifierSinaWeibo; NSString * const ACAccountTypeIdentifierTencentWeibo; NSString * const ACAccountTypeIdentifierLinkedIn;
|
在调用requestAccessToAccountsWithType方法时,可以传入一个字典参数,某些社交平台的授权需要配置一些额外参数,例如:
1 2 3 4 5 6 7 8 9 10 11 12
| NSString * const ACFacebookAppIdKey; NSString * const ACFacebookPermissionsKey; NSString * const ACFacebookAudienceKey; NSString * const ACFacebookAudienceEveryone; NSString * const ACFacebookAudienceFriends; NSString * const ACFacebookAudienceOnlyMe;
NSString * const ACLinkedInAppIdKey; NSString * const ACLinkedInPermissionsKey;
NSString *const ACTencentWeiboAppIdKey;
|
三、用户信息相关类解析
ACAccount类解析如下:
1 2 3 4 5 6 7 8 9
| @interface ACAccount : NSObject - (instancetype)initWithAccountType:(ACAccountType *)type; @property (readonly, weak, NS_NONATOMIC_IOSONLY) NSString *identifier; @property (strong, NS_NONATOMIC_IOSONLY) ACAccountType *accountType; @property (copy, NS_NONATOMIC_IOSONLY) NSString *accountDescription; @property (copy, NS_NONATOMIC_IOSONLY) NSString *username; @property (readonly, NS_NONATOMIC_IOSONLY) NSString *userFullName; @property (strong, NS_NONATOMIC_IOSONLY) ACAccountCredential *credential; @end
|
ACAccountCredential类解析如下:
1 2 3 4 5 6 7 8 9
| @interface ACAccountCredential : NSObject
- (instancetype)initWithOAuthToken:(NSString *)token tokenSecret:(NSString *)secret; - (instancetype)initWithOAuth2Token:(NSString *)token refreshToken:(NSString *)refreshToken expiryDate:(NSDate *)expiryDate;
@property (copy, nonatomic) NSString *oauthToken; @end
|
四、ACAccountStore类解析
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| @interface ACAccountStore : NSObject
@property (readonly, weak, NS_NONATOMIC_IOSONLY) NSArray *accounts;
- (ACAccount *)accountWithIdentifier:(NSString *)identifier;
- (ACAccountType *)accountTypeWithAccountTypeIdentifier:(NSString *)typeIdentifier;
- (NSArray *)accountsWithAccountType:(ACAccountType *)accountType;
- (void)saveAccount:(ACAccount *)account withCompletionHandler:(ACAccountStoreSaveCompletionHandler)completionHandler;
- (void)requestAccessToAccountsWithType:(ACAccountType *)accountType withCompletionHandler:(ACAccountStoreRequestAccessCompletionHandler)handler NS_DEPRECATED(NA, NA, 5_0, 6_0); - (void)requestAccessToAccountsWithType:(ACAccountType *)accountType options:(NSDictionary *)options completion:(ACAccountStoreRequestAccessCompletionHandler)completion;
- (void)renewCredentialsForAccount:(ACAccount *)account completion:(ACAccountStoreCredentialRenewalHandler)completionHandler;
- (void)removeAccount:(ACAccount *)account withCompletionHandler:(ACAccountStoreRemoveCompletionHandler)completionHandler; @end
|
千里之遥始于足下,冰冻三尺非一日之寒
——共勉